NO.1162 地图分析 中等
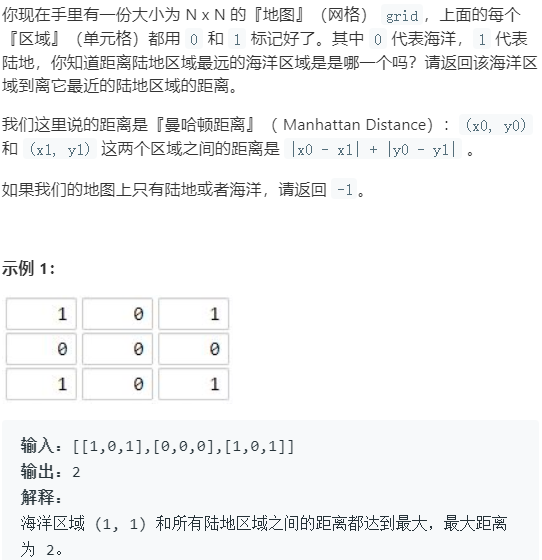
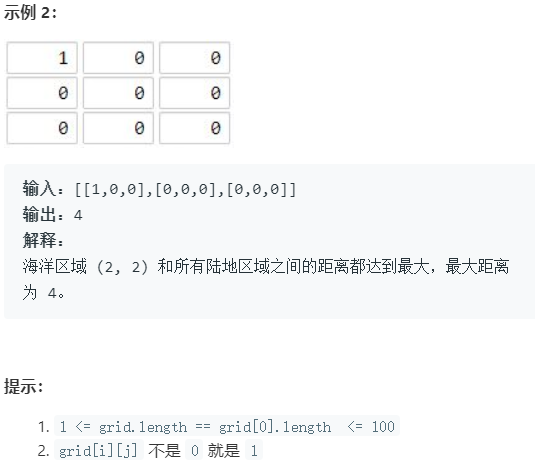
思路一:BFS BFS经常用来解决最短路径问题,但是本题用来求解最长路径问题也是可行的。
BFS求最短路径问题就是遇到第一个符合要求的节点就返回。
BFS求最长路径问题则是搜索完全部节点之后再返回。
对本题而言,先遍历将所有的陆地入队作为第0层,然后第0层出队放入第1层。。。广搜层序遍历。。。搜索结束时的层数就是陆地距离最远的海洋的距离。
用boolean数组标记网格的每个点是否入队过。或者”沉岛思想”直接在网格上进行标记,入过队的海洋(0)置为1(非0)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| public int maxDistance(int[][] grid) { int n = grid.length; Queue<int[]> queue=new ArrayDeque<>(); for (int i = 0; i < n; i++) { for (int j = 0; j < n; j++) { if (grid[i][j] == 1) { queue.add(new int[]{i,j}); } } } if (queue.size() == n * n || queue.isEmpty()) { return -1; } int round=-1; while (!queue.isEmpty()) { round++; int size = queue.size(); for (int i = 0; i < size; i++) { int[] temp = queue.poll(); if (temp[0] + 1 < n && grid[temp[0] + 1][temp[1]] == 0) { grid[temp[0] + 1][temp[1]] = 1; queue.add(new int[]{temp[0] + 1, temp[1]}); } if (temp[1] + 1 < n && grid[temp[0]][temp[1]+1] == 0) { grid[temp[0]][temp[1]+1]=1; queue.add(new int[]{temp[0],temp[1]+1}); } if (temp[0] - 1 >= 0 && grid[temp[0]-1][temp[1]] == 0) { grid[temp[0]-1][temp[1]] =1; queue.add(new int[]{temp[0]-1,temp[1]}); } if (temp[1] - 1 >= 0 && grid[temp[0]][temp[1]-1] == 0) { grid[temp[0]][temp[1]-1]=1; queue.add(new int[]{temp[0],temp[1]-1}); } } } return round; }
|
时间复杂度:O(n^2) 遍历一遍grid。
本人菜鸟,有错误请告知,感激不尽!
更多题解和源码:github